Pinia: vue3状态管理
一、Pinia 的相关介绍
1. 什么是 Pinia
Pinia 是一个专门配合 vue.js 使用的状态管理, 从而实现跨组建通信或实现多个组件共享数据的一种技术
2. 使用 Pinia 的目的
我们的vuejs有俩个特性: 1> 数据驱动视图. 2> 组件化开发
基于这俩个特性, 我们引出pinia的使用目的
1> 多个组件数据保持同步(一个组件改变数据, 其他的相关组件数据也发生变化)
2> 数据的变化是可追踪的(谁改了谁负责)
3. Pinia 中存什么?
多个组件共享的数据( 公共数据), 而某个组件中的私有数据, 依然存储在组件自身内部
比如:
• 登陆的用户名需要在首页、个⼈中心页、搜索页、商品详情页 使用, 则用户名存储在Pinia中
• 新闻详情数据, 只有在新闻详情页查看, 则存储在组件自身内部
二、Pinia 的使用
我们使用一个案例来进行学习
◦ 需求1: App.vue(作为根组件)
◦ 需求2: ⼦组件Add和⼦组件Sub, 作为在App.vue的⼦组件
◦ 需求3: 三个组件共享库存数据(保持同步)
准备工作:
准备App.vue, Add.vue,Sub.vue
我们先把环境准备好:
1. 4个固定步骤
1> 下包: npm i pinia
2> 导入: import { createPinia } from 'pinia'
3> 创建: const pinia = createPinia()
4> 注册: app.use(pinia)
2. 定义仓库
1> 导入定义仓库的函数
import {defineStore} from 'pinia'
2> 创建仓库, 并导出
export const useXxxStore defineStore(仓库id,Setup函数或Option对象)
3. 使用仓库
import { useXxxStored } from '仓库模块的相对路径'
const XxxStore = useXxxStore()
进而通过XxxStore调用仓库提供的数据和修改方法
4. 关于 defineStore('仓库id',函数或对象) 的注意:
1> 仓库 id 必须唯一
2> defineStore的返回值推荐使用 useXxxStore 来命名
3> defineStore的第二个参数支持函数或者对象
1) 函数传参: 本质还是vue3的写法, ref 或者 reactive 提供响应式数据, computed计算或者新数据, 修改数据直接定义函数, 最终要把这些数据和函数返回即可
2) 对象传参: 对象中包含的几个选项
1. state: 提供共享的数据
2. actions: 修改共享数据的函数
3. getters: 基于state计算得到新数据
函数传参
Add.vue 使用 addStock() 数据++
Sub.vue 使用 subStock() 数据--
App.vue
计算库存的双倍值
对象传参
具体的代码
main.js
// 按需导入 createApp 函数
import { createApp } from 'vue'
// 导入 App.vue
import App from './App.vue'
// 导入pinia
import { createPinia } from 'pinia'
// 创建应用
const pinia = createPinia()
const app = createApp(App)
//注册
app.use(pinia)
app.mount('#app')
stock.js
// 导入定义仓库的函数
import { defineStore } from "pinia";
import { ref, computed } from 'vue'
// 定义仓库
// defineStore('仓库的id名称',函数或对象)
// 函数传参: 类似于和Vue3的组合式API
// export const useStockStore = defineStore('stock', () => {
// // 计算 stock 的俩倍值: 使用 computed 计算属性
// const doubleStock = computed(() => {
// return stock.value * 2;
// })// // 提供共享数据
// const stock = ref(100)
// // 修改共享数据// // 新增
// function addStock() {
// stock.value++;
// }
// // 减少
// function subStock() {
// stock.value--;
// }
// // 返回共享数据和修改函数
// return {
// stock,
// addStock,
// subStock,
// doubleStock
// }
// })
// 对象传参: Option Store
export const useStockStore = defineStore('stock', {//state: 存放共享数据, 类似于 Setup 传参的 ref 或 reactivestate: () => ({stock: 100}),//actions: 存放修改数据的函数, 类似于 Setup 传参时修改数据的函数actions: {//新增库存addStock() {this.stock++;},//库存减少subStock() {this.stock--;}},//getters: 存放计算属性,类似于 computed 此时存放的是计算库存的俩倍值getters: {doubleStock: (state) => state.stock * 2}
})
Add.vue
<script setup>
// 按需导入 useStockStore 函数
import { useStockStore } from '@/store/stock';
// 调用函数, 获取仓库
const stockStore = useStockStore()
// console.log(stockStore)
</script><template><div class="add"><h3>Add组件</h3><p>已知库存数: {{ stockStore.stock }}</p><button @click="stockStore.addStock()">库存+1</button></div>
</template><style scoped></style>
Sub.vue
<script setup>
// 导入 useStockStore 函数
import { useStockStore } from '@/store/stock';
// 获取库存仓库
const stockStore = useStockStore();
</script><template><div class="sub"><h3>Sub组件</h3><p>已知库存数: {{ stockStore.stock }}</p><button @click="stockStore.subStock()">库存-1</button></div>
</template><style scoped></style>
App.vue
<script setup>
import Add from './components/Add.vue';
import Sub from './components/Sub.vue';
import { useStockStore } from './store/stock';
const stockStore = useStockStore()
// console.log(stockStore.getters.doubleStock)
</script>
<template><div><h1>根组件</h1><!-- 使用v-model.number 实现双向数据绑定的同时,指定里面的是数字, type里面改成number表示里面只能输入数字 -->库存总数: <input type="number" v-model.number="stockStore.stock"><p>stock 的翻倍值 ={{ stockStore.doubleStock }}</p><hr/><Add></Add><hr/><Sub></Sub></div>
</template><style>
#app{width: 350px;margin: 100px auto;padding: 5px 10px;border: 1px solid #ccc;
}
</style>
三、Pinia 重构代办任务案例
效果:
1. 使用组件化开发的思想, 搭建页面
1> 准备TodoHeader, TodoMain, TodoFooter 3 个组件
2> 在 App.vue 进行组装, 展示出正常的界面
把待办任务列表放在Pinia实现数据共享
2. Pinia的使用步骤
1> 4个固定步骤: 下包, 导入, 创建, 注册
2> 定义仓库
export const useTodoStore = defineStore('todo',{
state: () =>({todos:[]}),
getters{},
actions:{}
})
3> 使用仓库
1) 导入仓库
2) 得到仓库
3) 通过仓库获取数据或调用方法
3. 添加各种功能:
新增功能:
1> 在 todo 模块中, 定义添加方法
2> 在 TodoHeader 组件中, 调用添加方法, 传入参数
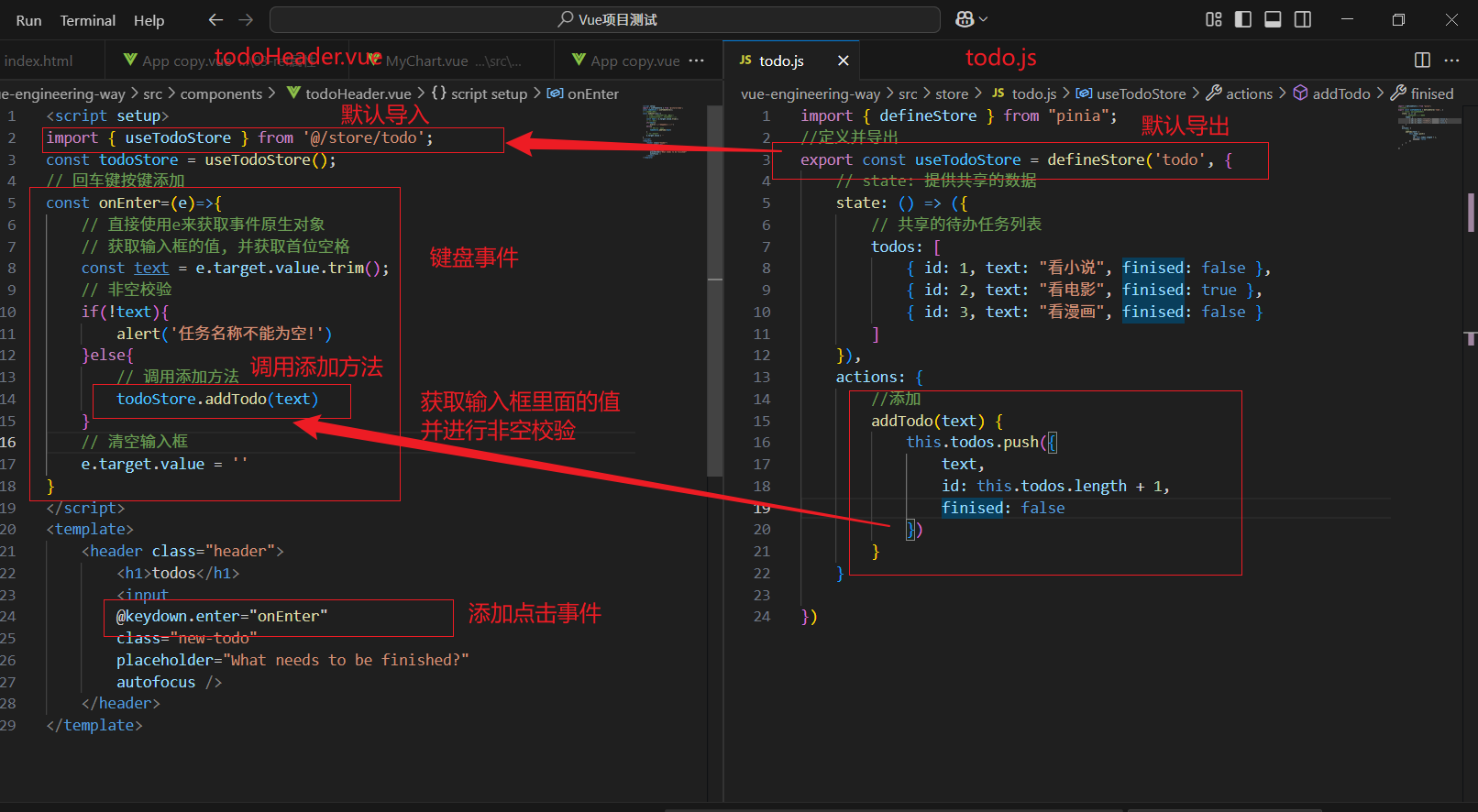
删除功能
1> 在 todo 模块中, 定义删除方法
2> 在 TodoMain 组件, 调用删除方法, 传入下标
小选全选功能
1. 小选全部选中, 全选需要选中; 否则全选不选中
1> 在 todo 模块中, 定义getters, 基于 todos 数组计算得到新的布尔值
2> 在 TodoMain组件中, 新定义一个计算属性isAllSelected,利用计算属性的完整写法: get+set
2. 全选选中, 小选全部选中; 否则小选都不选中
1> 利用 isAllSelected 的 set 函数, 接收全选的选中状态
2> 在 todo 模块中, 定义切换状态的函数, 遍历数组赋值即可
底部统计和清除功能
1. 底部统计: 显示几条未完成的任务
1> 在 todo 模块中, 计算数组中有几个对象的 finished 为 false, 定义 getters
2> 在 TodoFooter 组件中, 使用计算属性
2. 清除所有已完成
1> 在todo模块中, 定义清除已完成todo的方法
2> 在TodoFooter组件中, 调用清除方法
底部点击高亮和数据切换
1. 点击高亮
核心: 控制 a 标签动态绑定 selected 类
1> 在 todo 模块中, 定义一个数据, 积累当前选择类型, 一共有3种状态, 我们分别记录为: all, unfinished, finished
2> 动态的给 a 标签绑定 class, 并绑定点击事件,设置选择类型数据
2. 数据切换
1> 在todo模块中, 基于 type 和 todos 俩个已有的数据计算出需要展示的数据, 定义getters
2> 在TodoMain 组件中, 基于计算出的数据渲染列表
刚刚演示的是对象传参, 下面我们改写成函数传参
具体代码:
main.js
// 按需导入 createApp 函数
import { createApp } from 'vue'
// 导入 App.vue
import App from './App.vue'
// 导入pinia
import { createPinia } from 'pinia'
// 创建应用
const pinia = createPinia()
const app = createApp(App)
//注册
app.use(pinia)
app.mount('#app')
todo.js
import { defineStore } from "pinia";
import { ref, computed } from "vue";
// 记录选中的类型
// export const TODO_TYPE = {
// all: 'all', // 所有
// unfinished: 'unfinished', //未完成
// finished: 'finished' //已完成// }
//定义并导出, 对象传参
// export const useTodoStore = defineStore('todo', {
// // state: 提供共享的数据
// state: () => ({
// // 记录当前选择的类型, 默认值是all
// type: TODO_TYPE.all,
// // 共享的待办任务列表
// todos: [
// { id: 1, text: "看小说", finised: false },
// { id: 2, text: "看电影", finised: true },
// { id: 3, text: "看漫画", finised: false }
// ]
// }),
// //是否小选全部选中
// getters: {
// isAll: (state) => {
// return state.todos.every(item => item.finised)
// },
// //计算出所有未完成的todos
// unfinishedTodos: (state) => state.todos.filter((item) => !item.finised),
// //计算需要展示的todos
// showTodos: (state) => {
// //判断
// switch (state.type) {
// case TODO_TYPE.all:
// //返回所有数据
// return state.todos
// case TODO_TYPE.unfinished:
// //返回所有未完成的
// return state.todos.filter((item) => item.finised === false)
// case TODO_TYPE.finished:
// //返回所有已完成的
// return state.todos.filter((item) => item.finised)
// }
// }
// },
// //定义修改数据的函数
// actions: {
// //添加
// addTodo(text) {
// this.todos.push({
// text,
// id: this.todos.length + 1,
// finised: false
// })
// },
// //删除
// delTodo(i) {
// if (window.confirm('确认删除吗')) {
// this.todos.splice(i, 1)
// }
// },
// //切换状态
// toggleTodo(flag) {
// this.todos.forEach((item) => (item.finised = flag))
// },
// //清除已完成
// clearTodo() {
// //返回未完成的, 然后进行重新赋值
// this.todos = this.todos.filter(item => !item.finised)
// },
// // 设置类型
// setType(type) {
// this.type = type
// }
// }// })
// 定义并导出,
// 选中的类型
export const TODO_TYPE = {all: 'all', // 所有unfinished: 'unfinished', // 未完成finished: 'finished' // 已完成
}
// 定义并导出
export const useTodoStore = defineStore('todo', () => {// todos 数组const todos = ref([{ id: 1, text: 'Buy milk', finished: false },{ id: 2, text: 'Buy eggs', finished: true },{ id: 3, text: 'Buy bread', finished: false }])// 选择的类型const type = ref(TODO_TYPE.all)// 小选是否全部选中const isAll = computed(() => {return todos.value.every((item) => item.finished)})// 所有未完成的todosconst unfinishedTodos = computed(() => {return todos.value.filter((item) => !item.finished)})// 计算需要展示的todosconst showTodos = computed(() => {switch (type.value) {case TODO_TYPE.all:return todos.valuecase TODO_TYPE.unfinished:return todos.value.filter((item) => !item.finished)case TODO_TYPE.finished:return todos.value.filter((item) => item.finished)}})// 添加const addTodo = (text) => {todos.value.push({text,id: todos.value.length + 1,finished: false})}// 删除const delTodo = (i) => {if (window.confirm('确认删除么')) {todos.value.splice(i, 1)}}// 切换状态const toggleTodo = (flag) => {todos.value.forEach((item) => (item.finished = flag))}// 清除const clearTodo = () => {todos.value = todos.value.filter((item) => !item.finished)}// 设置选中类型const setType = (selectType) => {type.value = selectType}return {todos,type,isAll,unfinishedTodos,showTodos,addTodo,delTodo,toggleTodo,clearTodo,setType}
})
todoFooter.vue
<script setup>
import { useTodoStore,TODO_TYPE } from '@/store/todo';
const todoStore = useTodoStore()
</script>
<template><footer class="footer"><span class="todo-count"><strong>{{ todoStore.unfinishedTodos.length }}</strong> item left</span><ul class="filters"><li><a@click="todoStore.setType(TODO_TYPE.all)" href="#/" :class="{selected:todoStore.type === TODO_TYPE.all}">All</a></li><li><a @click="todoStore.setType(TODO_TYPE.unfinished)"href="#/active":class="{selected:todoStore.type === TODO_TYPE.unfinished}">Active</a></li><li><a @click="todoStore.setType(TODO_TYPE.finished)":class="{selected:todoStore.type === TODO_TYPE.finished}"href="#/completed">Completed</a></li></ul><!-- 删除已经完成的 --><button class="clear-completed"@click="todoStore.clearTodo()">Clear completed</button></footer>
</template>
todoHeader.vue
<script setup>
import { useTodoStore } from '@/store/todo';
const todoStore = useTodoStore();
// 回车键按键添加
const onEnter=(e)=>{// 直接使用e来获取事件原生对象// 获取输入框的值, 并获取首位空格const text = e.target.value.trim();// 非空校验if(!text){alert('任务名称不能为空!')}else{// 调用添加方法todoStore.addTodo(text)}// 清空输入框e.target.value = ''
}
</script>
<template><header class="header"><h1>todos</h1><input @keydown.enter="onEnter"class="new-todo" placeholder="What needs to be finished?" autofocus /></header>
</template>
todoMain.vue
<script setup>
// 导入 useTodoStore 函数
import { useTodoStore } from '@/store/todo';
//
import { computed } from 'vue';
// 获取仓库
const todoStore = useTodoStore()
// 计算属性: 小选和全选
const isAllSelected = computed({//把 isAll再包装一层, 这样计算属性就能既写get又写setget(){//获取自动触发, 必须有返回值return todoStore.isAll},// 赋值自动触发, 接收要赋予的新值set(flag){todoStore.toggleTodo(flag);}
})
// console.log(useTodoStore())
</script>
<template><section class="main"><inputv-model="isAllSelected" id="toggle-all" class="toggle-all" type="checkbox" /><label for="toggle-all">Mark all as complete</label><ul class="todo-list"><!-- 为ture 才会把这个类名加上 --><li v-for="(item,index) in todoStore.showTodos":key="item.id":class="{completed:item.finised}"><div class="view"><input v-model="item.finised"class="toggle" type="checkbox" /><label>{{ item.text }}</label><button class="destroy" @click="todoStore.delTodo(index)"></button></div></li></ul></section>
</template>
App.vue
<script setup>
//导入样式文件
import './assets/style.css'
//导入三个组件
import todoFooter from './components/todoFooter.vue';
import todoHeader from './components/todoHeader.vue';
import todoMain from './components/todoMain.vue';
</script>
<template><section class="todoapp"><todo-header /><todo-main /><todo-footer /></section>
</template>
style.css
@charset 'utf-8';html,
body {margin: 0;padding: 0;
}button {margin: 0;padding: 0;border: 0;background: none;font-size: 100%;vertical-align: baseline;font-family: inherit;font-weight: inherit;color: inherit;-webkit-appearance: none;appearance: none;-webkit-font-smoothing: antialiased;-moz-osx-font-smoothing: grayscale;
}body {font: 14px 'Helvetica Neue', Helvetica, Arial, sans-serif;line-height: 1.4em;background: #f5f5f5;color: #111111;min-width: 230px;max-width: 550px;margin: 0 auto;-webkit-font-smoothing: antialiased;-moz-osx-font-smoothing: grayscale;font-weight: 300;
}.hidden {display: none;
}.todoapp {background: #fff;margin: 130px 0 40px 0;position: relative;box-shadow: 0 2px 4px 0 rgba(0, 0, 0, 0.2), 0 25px 50px 0 rgba(0, 0, 0, 0.1);
}.todoapp input::-webkit-input-placeholder {font-style: italic;font-weight: 400;color: rgba(0, 0, 0, 0.4);
}.todoapp input::-moz-placeholder {font-style: italic;font-weight: 400;color: rgba(0, 0, 0, 0.4);
}.todoapp input::input-placeholder {font-style: italic;font-weight: 400;color: rgba(0, 0, 0, 0.4);
}.todoapp h1 {position: absolute;top: -100px;width: 100%;font-size: 50px;font-weight: 200;text-align: center;color: #b83f45;-webkit-text-rendering: optimizeLegibility;-moz-text-rendering: optimizeLegibility;text-rendering: optimizeLegibility;
}.new-todo,
.edit {position: relative;margin: 0;width: 100%;font-size: 24px;font-family: inherit;font-weight: inherit;line-height: 1.4em;color: inherit;padding: 6px;border: 1px solid #999;box-shadow: inset 0 -1px 5px 0 rgba(0, 0, 0, 0.2);box-sizing: border-box;-webkit-font-smoothing: antialiased;-moz-osx-font-smoothing: grayscale;
}.new-todo {padding: 16px 16px 16px 60px;height: 65px;border: none;background: rgba(0, 0, 0, 0.003);box-shadow: inset 0 -2px 1px rgba(0, 0, 0, 0.03);
}.main {position: relative;z-index: 2;border-top: 1px solid #e6e6e6;
}.toggle-all {width: 1px;height: 1px;border: none; /* Mobile Safari */opacity: 0;position: absolute;right: 100%;bottom: 100%;
}.toggle-all + label {display: flex;align-items: center;justify-content: center;width: 45px;height: 65px;font-size: 0;position: absolute;top: -65px;left: -0;
}.toggle-all + label:before {content: '❯';display: inline-block;font-size: 22px;color: #949494;padding: 10px 27px 10px 27px;-webkit-transform: rotate(90deg);transform: rotate(90deg);
}.toggle-all:checked + label:before {color: #484848;
}.todo-list {margin: 0;padding: 0;list-style: none;
}.todo-list li {position: relative;font-size: 24px;border-bottom: 1px solid #ededed;
}.todo-list li:last-child {border-bottom: none;
}.todo-list li.editing {border-bottom: none;padding: 0;
}.todo-list li.editing .edit {display: block;width: calc(100% - 43px);padding: 12px 16px;margin: 0 0 0 43px;
}.todo-list li.editing .view {display: none;
}.todo-list li .toggle {text-align: center;width: 40px;/* auto, since non-WebKit browsers doesn't support input styling */height: auto;position: absolute;top: 0;bottom: 0;margin: auto 0;border: none; /* Mobile Safari */-webkit-appearance: none;appearance: none;
}.todo-list li .toggle {opacity: 0;
}.todo-list li .toggle + label {/*Firefox requires `#` to be escaped - https://bugzilla.mozilla.org/show_bug.cgi?id=922433IE and Edge requires *everything* to be escaped to render, so we do that instead of just the `#` - https://developer.microsoft.com/en-us/microsoft-edge/platform/issues/7157459/*/background-image: url('data:image/svg+xml;utf8,%3Csvg%20xmlns%3D%22http%3A//www.w3.org/2000/svg%22%20width%3D%2240%22%20height%3D%2240%22%20viewBox%3D%22-10%20-18%20100%20135%22%3E%3Ccircle%20cx%3D%2250%22%20cy%3D%2250%22%20r%3D%2250%22%20fill%3D%22none%22%20stroke%3D%22%23949494%22%20stroke-width%3D%223%22/%3E%3C/svg%3E');background-repeat: no-repeat;background-position: center left;
}.todo-list li .toggle:checked + label {background-image: url('data:image/svg+xml;utf8,%3Csvg%20xmlns%3D%22http%3A%2F%2Fwww.w3.org%2F2000%2Fsvg%22%20width%3D%2240%22%20height%3D%2240%22%20viewBox%3D%22-10%20-18%20100%20135%22%3E%3Ccircle%20cx%3D%2250%22%20cy%3D%2250%22%20r%3D%2250%22%20fill%3D%22none%22%20stroke%3D%22%2359A193%22%20stroke-width%3D%223%22%2F%3E%3Cpath%20fill%3D%22%233EA390%22%20d%3D%22M72%2025L42%2071%2027%2056l-4%204%2020%2020%2034-52z%22%2F%3E%3C%2Fsvg%3E');
}.todo-list li label {overflow-wrap: break-word;padding: 15px 15px 15px 60px;display: block;line-height: 1.2;transition: color 0.4s;font-weight: 400;color: #484848;
}.todo-list li.completed label {color: #949494;text-decoration: line-through;
}.todo-list li .destroy {display: none;position: absolute;top: 0;right: 10px;bottom: 0;width: 40px;height: 40px;margin: auto 0;font-size: 30px;color: #949494;transition: color 0.2s ease-out;
}.todo-list li .destroy:hover,
.todo-list li .destroy:focus {color: #c18585;
}.todo-list li .destroy:after {content: '×';display: block;height: 100%;line-height: 1.1;
}.todo-list li:hover .destroy {display: block;
}.todo-list li .edit {display: none;
}.todo-list li.editing:last-child {margin-bottom: -1px;
}.footer {padding: 10px 15px;height: 40px;text-align: center;font-size: 15px;border-top: 1px solid #e6e6e6;
}.footer:before {content: '';position: absolute;right: 0;bottom: 0;left: 0;height: 50px;overflow: hidden;box-shadow: 0 1px 1px rgba(0, 0, 0, 0.2), 0 8px 0 -3px #f6f6f6,0 9px 1px -3px rgba(0, 0, 0, 0.2), 0 16px 0 -6px #f6f6f6,0 17px 2px -6px rgba(0, 0, 0, 0.2);
}.todo-count {float: left;text-align: left;
}.todo-count strong {font-weight: 300;
}.filters {margin: 0;padding: 0;list-style: none;position: absolute;right: 0;left: 0;
}.filters li {display: inline;
}.filters li a {color: inherit;margin: 3px;padding: 3px 7px;text-decoration: none;border: 1px solid transparent;border-radius: 3px;
}.filters li a:hover {border-color: #db7676;
}.filters li a.selected {border-color: #ce4646;
}.clear-completed,
html .clear-completed:active {float: right;position: relative;line-height: 19px;text-decoration: none;cursor: pointer;
}.clear-completed:hover {text-decoration: underline;
}.info {margin: 65px auto 0;color: #4d4d4d;font-size: 11px;text-shadow: 0 1px 0 rgba(255, 255, 255, 0.5);text-align: center;
}.info p {line-height: 1;
}.info a {color: inherit;text-decoration: none;font-weight: 400;
}.info a:hover {text-decoration: underline;
}/*Hack to remove background from Mobile Safari.Can't use it globally since it destroys checkboxes in Firefox
*/
@media screen and (-webkit-min-device-pixel-ratio: 0) {.toggle-all,.todo-list li .toggle {background: none;}.todo-list li .toggle {height: 40px;}
}@media (max-width: 430px) {.footer {height: 50px;}.filters {bottom: 10px;}
}:focus,
.toggle:focus + label,
.toggle-all:focus + label {box-shadow: 0 0 2px 2px #cf7d7d;outline: 0;
}